Listen for @bot mentions
Get notified when someone tags your bot in a cast
The easiest way to listen for when your bot gets tagged in a cast is to set up a webhook in the Neynar developer portal and integrate it into your bot codebase.
Set up a webhook for your bot on Neynar Dev portal
To create a new webhook without writing any code, head to the neynar dashboard and go to the webhooks tab.
Get events when your bot gets tagged in a cast e.g. @bot
@bot
Click on the new webhook and enter the fid
of your bot in the mentioned_fids
field for a cast.created
filter.
What this does is anytime a cast is created on the protocol, it checks if your bot that has that fid
is mentioned in the cast. If it is, it fires a webhook event to your backend.
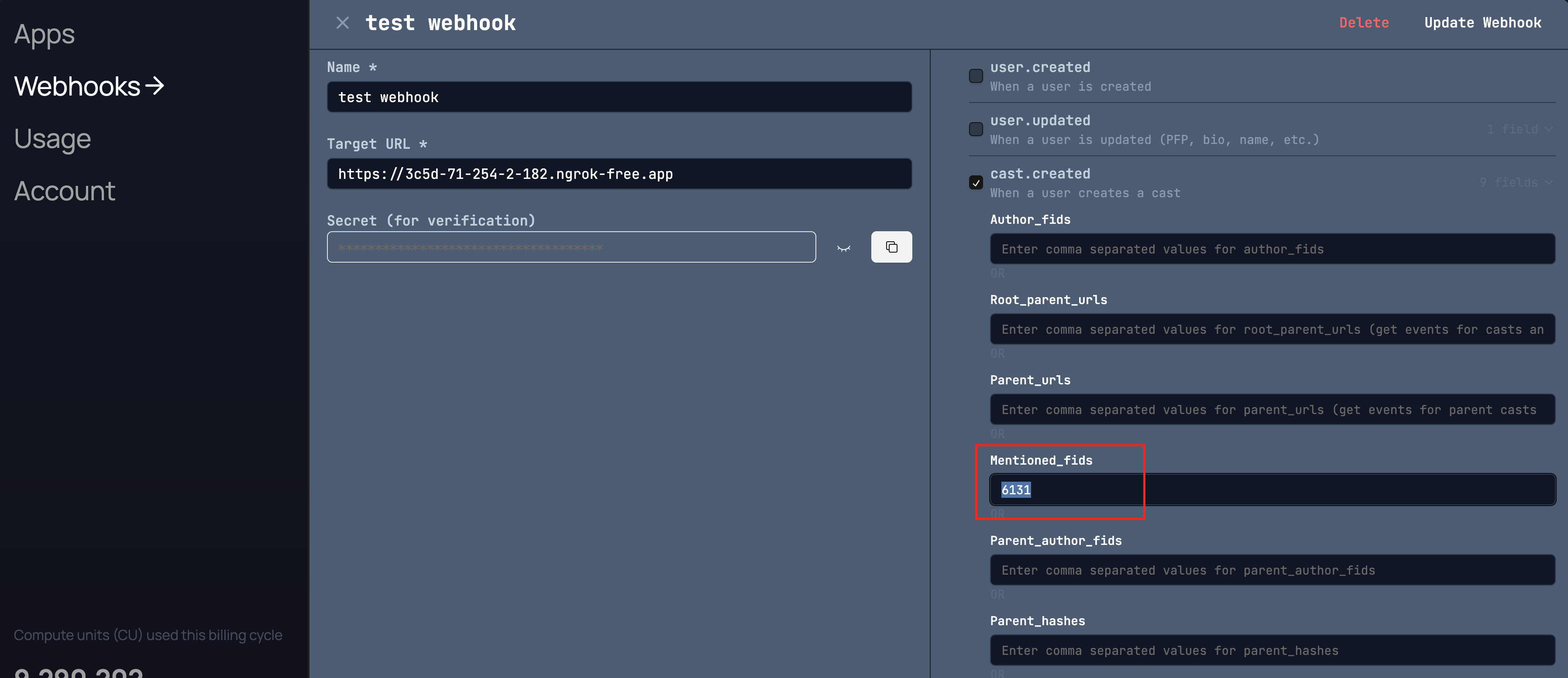
Get events when someone replies to your bot
In the same webhook as above, insert the fid
of your bot in the parent_author_fids
field. See screenshot below. This will fire an event for whenever someone casts a reply where your bot is the parent cast's author.
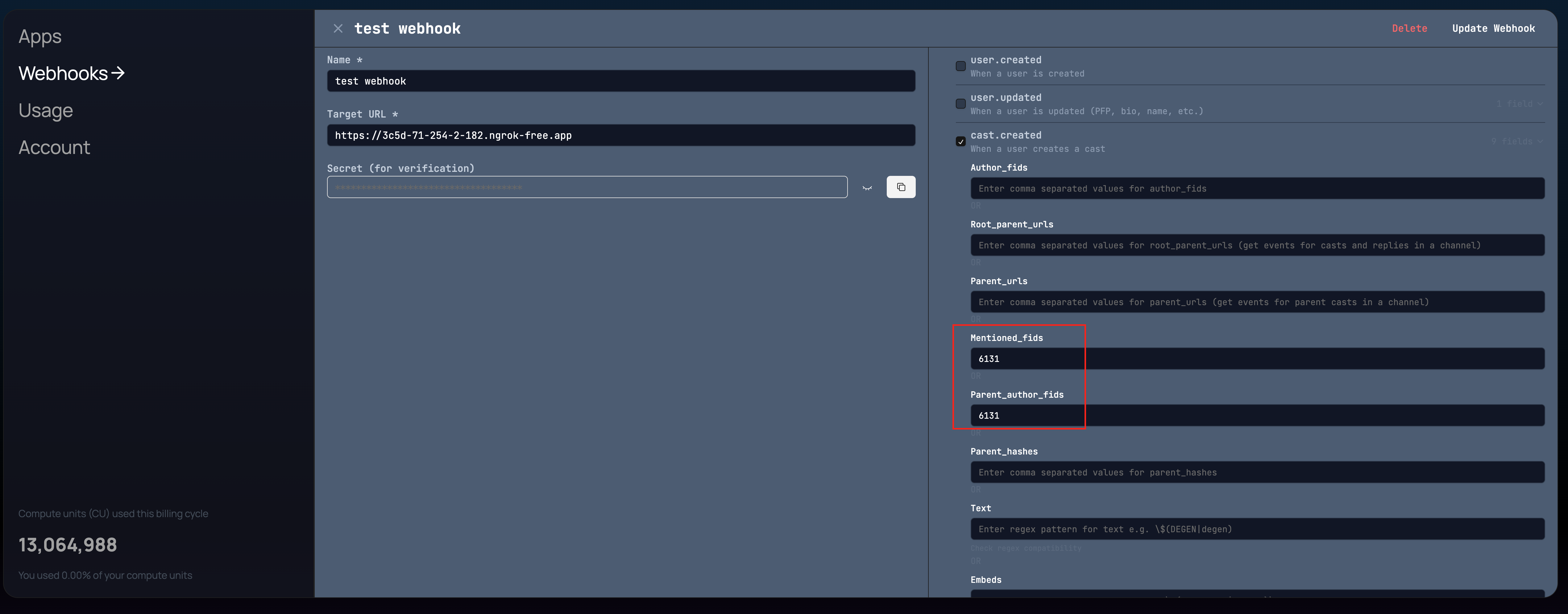
You will notice that the same webhook now has two filters for the cast.created
event. This is because webhook filters are logical OR
filters meaning that the event will fire if any one of the conditions are fulfilled. In this case, the webhook server will notify your backend if someone
- tags your bot i.e.
mentioned_fids
filter - replies to your bot i.e.
parent_author_fids
filter
Expand as needed
If you build more than one bot, you can continue adding those fids to these fields in comma separated format and you will get webhook events for any of the bots (or users, doesn't have to be bots).
Now let's move on to processing the events you receive on your backend.
Receive real time webhook events on your backend
Setting up a POST url
Your backend needs a POST url to listen for incoming webhook events. The webhook will fire to the specified target_url
. For the purpose of this demo, we used ngrok to create a public URL. You can also use a service like localtunnel that will forward requests to your local server. Note that free endpoints like ngrok, localtunnel, etc. usually have issues because service providers start blocking events. Ngrok is particularly notorious for blocking our webhook events. This is best solved by using a url on your own domain.
Server to process events received by the POST url
Let's create a simple server that logs out the event. We will be using Bun JavaScript.
const server = Bun.serve({
port: 3000,
async fetch(req) {
try {
console.log(await req.json());
return new Response("gm!");
} catch (e: any) {
return new Response(e.message, { status: 500 });
}
},
});
console.log(`Listening on localhost:${server.port}`);
Next: run bun serve index.ts
, and run ngrok with ngrok http 3000
. Copy the ngrok URL and paste it into the "Target URL" field in the Neynar developer portal. The webhook will call the target URL every time the selected event occurs. Here, I've chosen to receive all casts that mention @neynar
in the text by putting in @neynar's fid
: 6131.
Now the server will log out the event when it is fired. It will look something like this:
{
created_at: 1708025006,
type: "cast.created",
data: {
object: "cast",
hash: "0xfe7908021a4c0d36d5f7359975f4bf6eb9fbd6f2",
thread_hash: "0xfe7908021a4c0d36d5f7359975f4bf6eb9fbd6f2",
parent_hash: null,
parent_url: "chain://eip155:1/erc721:0xfd8427165df67df6d7fd689ae67c8ebf56d9ca61",
root_parent_url: "chain://eip155:1/erc721:0xfd8427165df67df6d7fd689ae67c8ebf56d9ca61",
parent_author: {
fid: null,
},
author: {
object: "user",
fid: 234506,
custody_address: "0x3ee6076e78c6413c8a3e1f073db01f87b63923b0",
username: "balzgolf",
display_name: "Balzgolf",
pfp_url: "https://i.imgur.com/U7ce6gU.jpg",
profile: [Object ...],
follower_count: 65,
following_count: 110,
verifications: [ "0x8c16c47095a003b726ce8deffc39ee9cb1b9f124" ],
active_status: "inactive",
},
text: "@neynar LFG",
timestamp: "2024-02-15T19:23:22.000Z",
embeds: [],
reactions: {
likes: [],
recasts: [],
},
replies: {
count: 0,
},
mentioned_profiles: [],
},
}
These events will be delivered real time to your backend as soon as they appear on the protocol. If you see a cast on a client but you haven't received it on your backend:
- make sure you're not using ngrok or a similar service, use your own domain.
- check the cast hash/url on https://explorer.neynar.com to see where it's propagated on the network. If it hasn't propagated to the hubs, the network doesn't have the cast and thus the webhook didn't fire.
- make sure your backend server is running to receive the events.
Once you receive the event, return a 200
success to the webhook server else it will keep retrying the same event delivery.
Create webhooks programmatically
Now that you know how to set up webhooks manually on the dev portal, you might be wondering how to create them dynamically. You can do so using our Webhook APIs. They will allow you to create, delete or update webhooks. Few things to remember when creating webhooks programmatically:
- You can add an almost infinite number of filters to the same webhook so no need to create new webhooks for new filters.
- Filters are overwritten with new filters. So for e.g. if you are listening to mentions of fid 1 and now you want to listen to mentions of fid 1 and 2, you should pass in both 1 and 2 in the filters when you update.
You're ready to build!
That's it, it's that simple! Make sure to sure what you built with us on Farcaster by tagging @neynar and if you have any questions, reach out to us on warpcast or Telegram!
Updated 2 months ago